next permutation in c++|c++ : Manila Permutes the range [first, last) into the next permutation. Returns true if such a “next permutation” exists; otherwise transforms the range into the lexicographically first . Fighting Cocks is Life. I was born with a chick on my head. I grew up surrounded by my family of afficionados, every gathering must have a family derby..
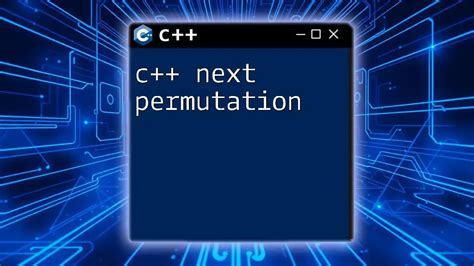
next permutation in c++,Examples: Input: N = 6, arr = {1, 2, 3, 6, 5, 4} Output: {1, 2, 4, 3, 5, 6} Explanation: The next permutation of the given array is {1, 2, 4, 3, 5, 6}. Input: N = 3, arr = {3, 2, 1} Output: {1, 2, 3} Explanation: As arr [] is the last permutation. So, the next .Implement the next permutation, which rearranges the list of numbers into .
std::next_permutation and prev_permutation in C++. Last Updated .
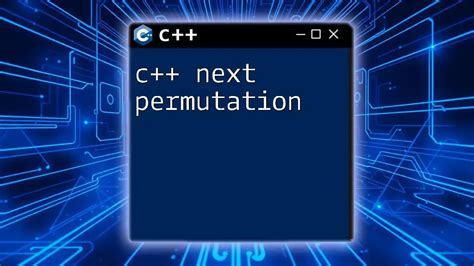
Permutes the range [first, last) into the next permutation. Returns true if such a “next permutation” exists; otherwise transforms the range into the lexicographically first . std::next_permutation and prev_permutation in C++. Last Updated : 01 Mar, 2023. std::next_permutation. It is used to rearrange the elements in the range [first, .template bool next_permutation(Iterator first, Iterator last) { if (first == last) return false; Iterator i = last; if (first == --i) return false; while (1) { Iterator i1 = i, i2; if (*--i < .// next_permutation example #include // std::cout #include // std::next_permutation, std::sort int main { int myints[] = {1,2,3}; std::sort .Implement the next permutation, which rearranges the list of numbers into Lexicographically next greater permutation of list of numbers. If such arrangement is .
Transforms the range [first, last) into the next permutation from the set of all permutations that are lexicographically ordered with respect to operator< or comp. Returns true if such .
std::next_permutation generates the next permutation in just linear time, and it can also handle repeated characters and generates distinct permutations. Its .Next Permutation - A permutation of an array of integers is an arrangement of its members into a sequence or linear order. * For example, for arr = [1,2,3], the following are all the . next_permutation. generates the next greater lexicographic permutation of a range of elements (function template) [edit] prev_permutation. generates the next smaller .
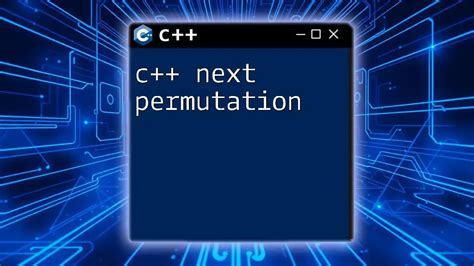
The algorithm. We will use the sequence (0, 1, 2, 5, 3, 3, 0) as a running example. The key observation in this algorithm is that when we want to compute the next permutation, we must “increase” the sequence as little as possible. Just like when we count up using numbers, we try to modify the rightmost elements and leave the left side . 1) Transforms the range [first, last) into the next permutation, where the set of all permutations is ordered lexicographically with respect to binary comparison function object comp and projection function object proj.Returns {last, true} if such a "next permutation" exists; otherwise transforms the range into the lexicographically first .
5. Yes, the simplest way is to override operator< within your class in which case you don't need to worry about comp. The comp parameter is a function pointer which takes two iterators to the vector and returns true or false depending on how you'd want them ordered. Edit: Untested but for what it's worth: myclass() : m_a( 0 ){} void operator .
The function is next_permutation(a.begin (), a.end ()) . It returns ‘true’ if the function could rearrange the object as a lexicographically greater permutation. Otherwise, the function returns ‘false’. Example: Time Complexity: O (N), where N is the length of the given string. Auxiliary Space: O (1), as constant extra space is used.
std::next_permutation returns the next permutation in lexicographic order, and returns false if the first permutation (in that order) is generated. Since the string you start with ( "xxxxxoooo") is actually the last permutation of that string's characters in lexicographic order, your loop stops immediately. Therefore, you may try sorting moves . これでも全く同じことができます。 4.prev_permutation. prev_permutationを使うとnext_permutationの逆のこと、つまり指定された配列(又はコンテナクラス)の順列を全て並べたときに、その配列を辞書順で前のものに置き換えることができます。 上でv={4,3,2,1}としてnext_permutationの代わりにprev_permutationを使 . This is a program which you can get the next "different", i.e. elements in A[] can be identical, permutation at each call. I've written a C++ program for the same purpose in 2018, but I found it unreadable, readability = NULL, so here is a new one. Any critical comment and answer will be welcomed :) next_different_permutation.c:c++ Download ZIP. Next permutation in C - CodeSignal: stringsRearrangement. Raw. next_permutation.c. This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters. Learn more about bidirectional Unicode .next permutation in c++C++ Algorithm next_permutation C++ Algorithm next_permutation() function is used to reorder the elements in the range [first, last) into the next lexicographically greater permutation.. A permutation is specified as each of several possible ways in which a set or number of things can be ordered or arranged. It is denoted as N! where N = number of .
C++ STL全排列 next_permutation 用法. 臭做游戏的。. 全排列是排列数学中常用的算法之一,而C++ STL中就提供了内置的全排列函数 next_permutation. 方法原型主要有以下两种(均以经过个人简化). next_permutation是一个原地算法(会直接改变这个集合,而不是返 . is_permutation:判斷陣列 b 是否為陣列 a 排序後的結果。 is_permutation(a, a+5, b); next_permutation:使用已經排序(由小到大)的資料,產生下一組排列。 prev_permutation:針對已經「逆向」排序(由大到小)的資料,產生上一組排序。 【範例】ZeroJudge e446: 排列生成The next_permutation() function is available under the header file in C++.. This function rearranges the elements into the next lexicographical a generalization of the alphabetical order of the dictionaries greater permutation possible ways in which a number of things can be reordered or arranged.. For example, if you have the elements [1, 2, 3], .Back to Explore Page. Implement the next permutation, which rearranges the list of numbers into Lexicographically next greater permutation of list ofnumbers. If such arrangement is not possible, it must be rearranged to the lowest possible order i.e.sorted in. Be aware that arrays in C don't know their length (that's why count must be passed), so if there's a mismatch between both arrays and/or size, your program will do something unexpected or crash. The are a lot of details to know about arrays and pointers in C. Please have a good look at this chapter of the famous C-FAQ.
next permutation in c++ c++ There are two overloads: template< class BidirIt >. bool next_permutation( BidirIt first, BidirIt last ); template< class BidirIt, class Compare >. bool next_permutation( BidirIt first, BidirIt last, Compare comp ); Neither of them matches your call. Use std::string::begin and std::string::end to get iterators to the range of characters inside . The lexicographic or lexicographical order (aka lexical order, dictionary order, alphabetical order) means that the words are arranged as they are presumed to appear in a dictionary. For example, the next permutation in lexicographic order for string 123 is 132. The STL provides std::next_permutation, which returns the next permutation in . A permutation also called an “arrangement number” or “order,” is a rearrangement of the elements of an ordered list S into a one-to-one correspondence with S itself. A string of length n has n! permutation. . Next. C Program for Reverse a linked list. Share your thoughts in the comments. Add Your Comment.It can be implemented using the next permutation algorithm, which rearranges the string into the lexicographically next greater permutation. Breadth-First Search (BFS) Approach: This method uses a queue to generate permutations in a breadth-first manner. Approach 1: Permutation of Strings in C Example Using Backtracking Method
next permutation in c++|c++
PH0 · std::ranges:: next
PH1 · std::next
PH2 · next
PH3 · c++
PH4 · Next Permutation